Get blogged
Well, look at that! A very informative blog!
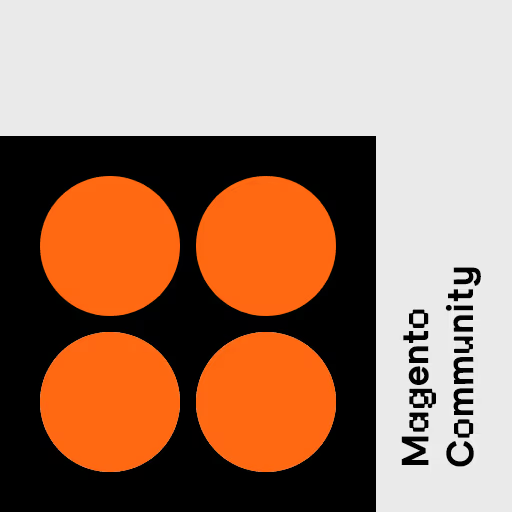
02/28/2025
Innovation and AI everywhere: Recap of E-Commerce Berlin Expo
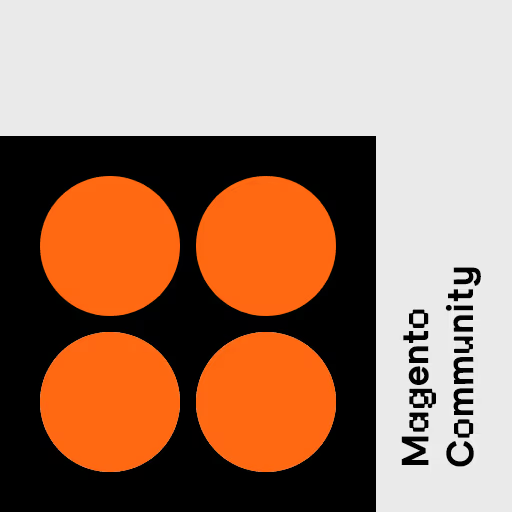
Community
11/06/2024
Recap of MageUnconf 2024
Magento 2
06/18/2024
Event-driven Architecture with Mage-OS or Magento 2
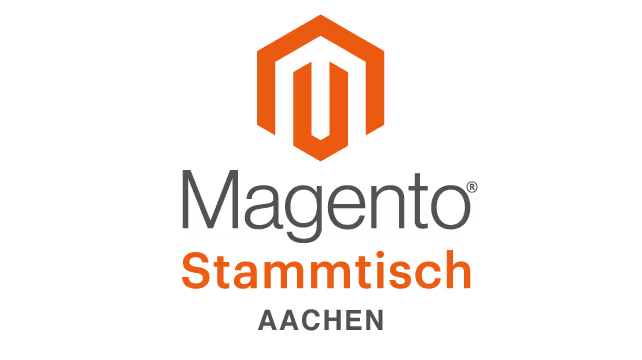
Community
05/24/2024
53. Magento Stammtisch Aachen
Security
02/15/2024
How we deployed security patches to 20 shops within less than a day
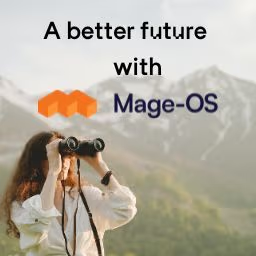
Magento 2
02/01/2024
A shop with Magento & Hyvä: a good investment in the future
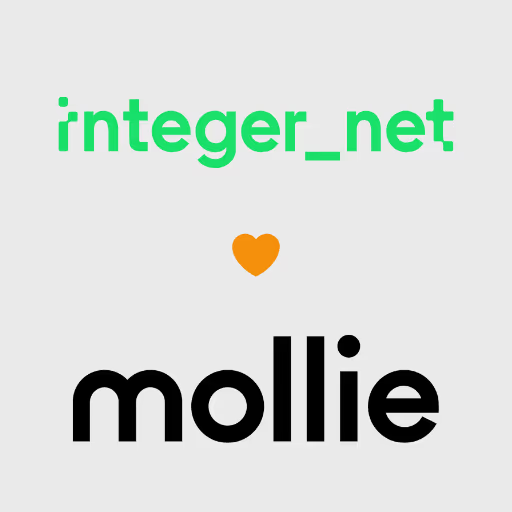
Magento 2
02/01/2024
Why we increasingly rely on Mollie as a payment provider
Community
12/20/2023
Magento Meetup Aachen: The Magento Community is strong - A recap
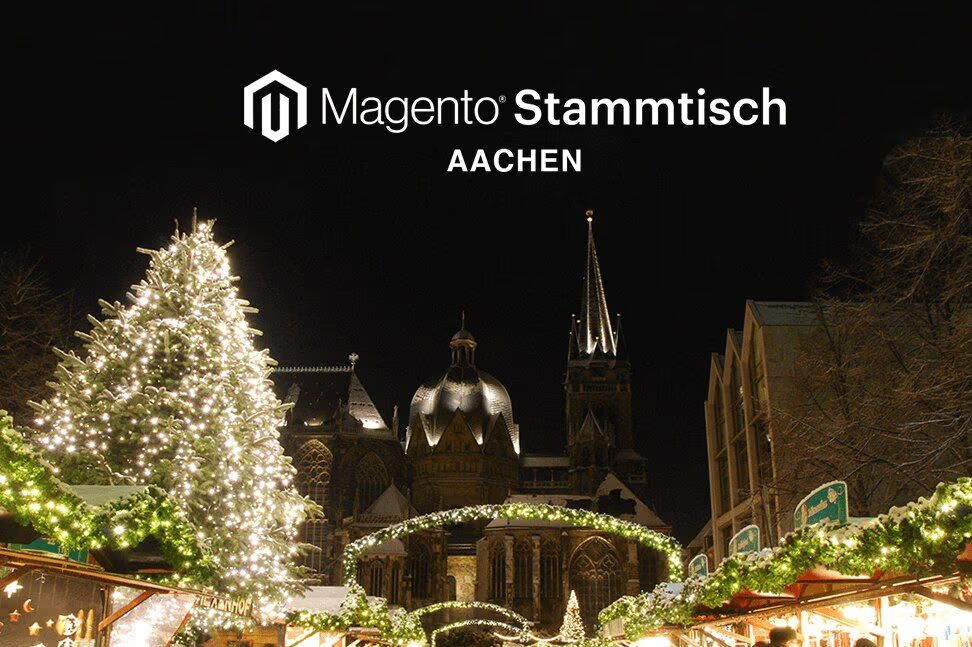
Community
10/20/2023
A very special Magento Meetup Aachen: The Christmas Edition
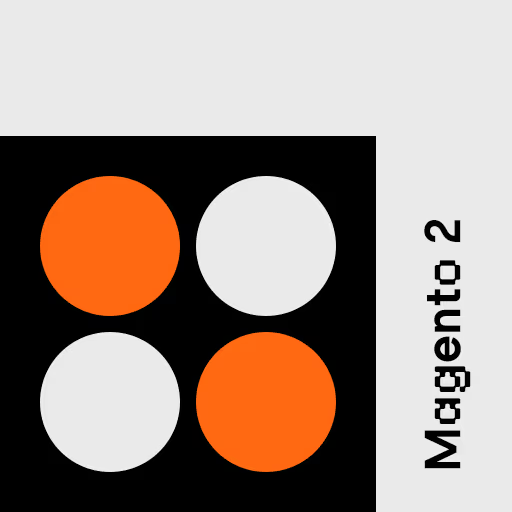
Magento 2
07/28/2023
Paypal Plus replaced by Paypal Checkout. What do I have to do?
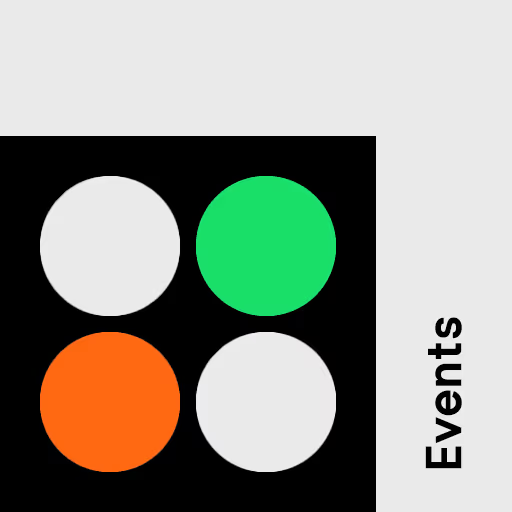
Community
10/06/2022
MageUnconference 2022 - Virality of Enthusiasm
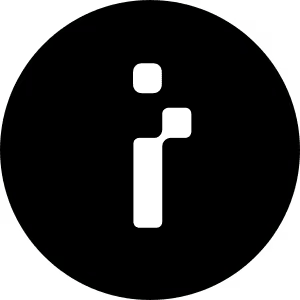
integer_net
09/16/2022
The new integer_net
Page 1 / 6